Last week I mentioned that I would be starting a new series of sorts. Today will be the first entry in that series. My hope is to bridge the gap between point and click development, and slinging code for those admins/developers that are hesitant to make “the leap of faith” into the world of of development. As mentioned, fellow MVP @SteveMoForce had mentioned that perhaps seeing a formula of sorts (be it from a validation rule, workflow entry criteria, etc) written out to its equivalent in Apex would be of use to begin understanding it.
While writing this stuff likely isn’t going to be useful to you directly, the intent is that since — as an admin — you are already familiar with how formulas work and how to write them that it isn’t as far a stretch to go from the logic of formulas to the logic of slinging code. After all, that is the biggest hurdle when learning to code, the logic. The rest is just syntax, like learning a new language, and for you the admin, more like learning the nuances of a given dialect of a language.
So for our first example, SteveMo was kind enough to donate the following formula that simply looks at four fields, determines if there is a value in any of them and if there is a value in more than one, return true. I’m told this was part of a validation rule the expects that “any” of the fields can be filled out, but only one of them can contain data at any given time:
IF(ISBLANK(Field_A), 0, 1) +
IF(ISBLANK(Field_B), 0, 1) +
IF(ISBLANK(Field_C), 0, 1) +
IF(ISBLANK(Field_D), 0, 1) > 1
What we have in this formula is a few “IF” functions, some math operations, and a comparison. (Comparisons always return true or false, since a Validation rule fires when its criteria is true, we need a comparison at the end of the day). Before jumping into the coding portion, lets ensure we all understand this formula.
IF(ISBLANK(Field_X), 0, 1):
When you use an IF function in a formula, it checks to find out if a given condition is true. Upon finding it is true it returns the next value in the “IF box”, if its false it returns the last value in the IF. So in this case we are checking to see if the value of the given field (Field_A) is blank. If it is indeed blank (condition is true) then the “value” in our IF box is 0, if it is not blank, the value of our IF box is 1.
We Do Math:
We take the results of these IFs and add them together, if they are all blank we get 0 + 0 + 0 + 0 = 0. If only one of the fields is filled out (it doesn’t matter which), we could get 0 + 0 + 1 + 0 = 1. Lastly if more than one field is filled out (again any combination) we could end up with 1 + 0 + 1 + 1 = 3.
Now we compare:
Finally we will look at the result of our math and check to see if it is greater than 1. If it is greater than one, our Validation Rule becomes TRUE and will fire a message. If it equals or is less than one, our Validation Rule is FALSE and all is well.
So let’s write some Apex:
I know your excited, but I want to clear a few things up. Firstly, we have to imagine for now that we have done some other coding to get access to this object’s fields. Following Steve’s example lets say we have a Widget object with the following four fields: Field_A__c, Field_B__c, Field_C__c and Field_D__c. Furthermore, as it is out of scope for this series, lets assume we’ve written the necessary code to allow us to refer these fields as “MyObject.Field_A__c” etc. Secondly, this code will be very verbose. There will likely be more concise/better ways to write this, but we have to walk before we run. Lastly, I may use words like variable or other “coding words” — I will try to explain those as I go, but remember the concept here is to map what you know of formulas to something you are unfamiliar with so that your mind can begin to marry the two together. This is largely an experiment so whether this helps remains to be seen. Stay with me.
Lets look at the code (I put the code in an image for two reasons. First, so that I get nice line numbers with my code and its easier to do a walkthrough. Second, so that if you wanted to play with this, you’d have to type it in manually. I know, I’m a jerk but cutting and pasting is one thing, but I believe it helps you absorb it all if you type it in as you go. That being said, this won’t compile directly in eclipse or sublime — remember that we are assuming I’ve done some other stuff, but perhaps some of the more enthusiastic amongst you might read up on that “other stuff” and I don’t want you mad at me when it doesn’t compile):
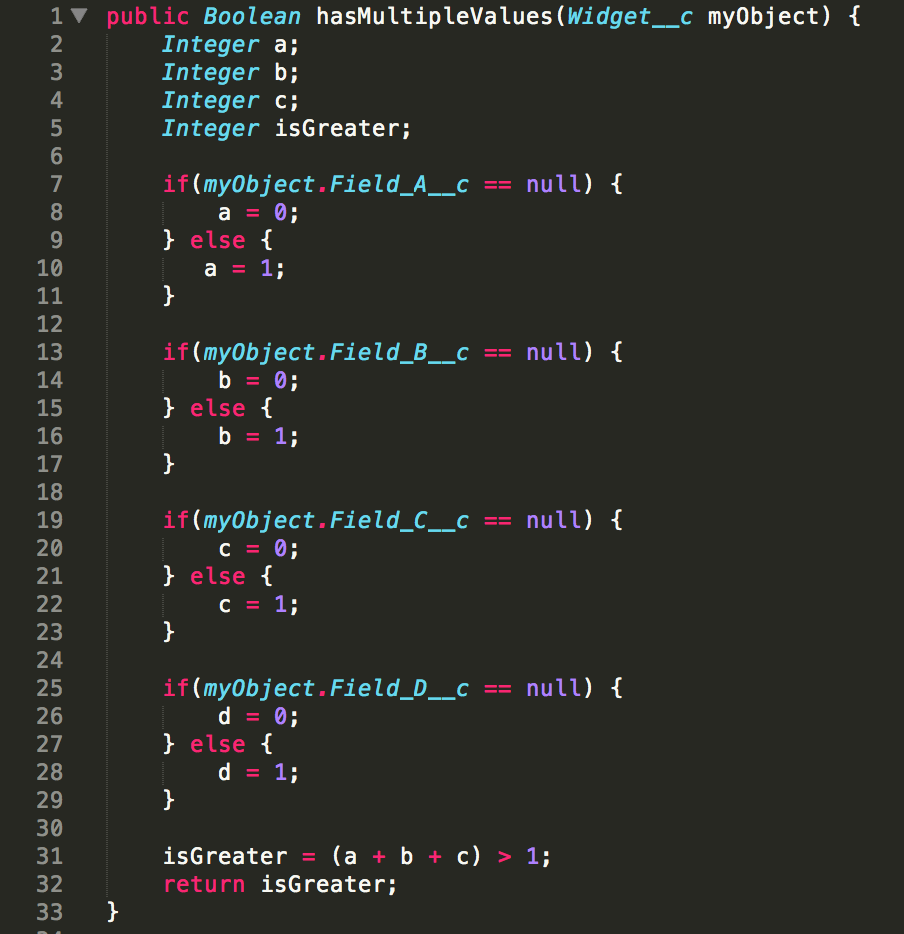
Now lemme ‘splain:
Line 1: Don’t get hung-up here. This is how we write a chunk of code that we can refer to later from some other place. Its called a function and we write code that “calls” it, which basically means, do the stuff in between my curly braces ({…}). For now just consider line 1 and line 33 as the walls of the container in which we are holding our apex version of the validation rule. The “black box” of magic so to speak.
Lines 2-4: Here we are telling the compiler (the bit that checks to make sure our code is valid and will run) that we are going to use some variables to hold integer values (whole numbers, not decimals, not text). Variables are placeholders for items we want to refer back to later, or do something with. In our case, we will be putting a value of either 0 or 1 in each of these based on whether or not our fields have a value in them and adding them up later.
Line 5: Here we are telling the compiler about another variable, but instead of holding a number it will hold a Boolean value which simply put, means its going to hold a value of TRUE or FALSE.
Line 7-11:
This might look familiar, its an IF statement quite similar to the IF function used in our formula. There is a certain syntax wrapped around us here so now would be a good time to point that out. An IF statement in Apex has a condition that you are checking for in parenthesis (in this scenario, the code in between the parenthesis is similar to the ISBLANK portions of our Validation Rule criteria), and then some curly braces. The curly braces separate the logic for us a bit. The code in between the first set of curly braces are evaluated when the condition in parenthesis is true. An if statement may also have more sets of curly braces. In our case we have an “else {…}” block. THIS block gets evaluated when our condition is not true (and therefore false, get it?). At some point in the future we can talk about the “else if {…}” block which allows us to check for numerous conditions by stringing them together, until one of the conditions finally winds up true or we run into an else block, or just run out of conditions to check all together.
So continuing with line 7-11, on line 7 we are checking our condition: if(myObject.Field_A__c == null). What is null you ask? Null is essentially “nothing” — so we are checking to see if there is any value there or not. The check for equality in Apex is the double equal sign “==” so this bit is equivalent to our ISBLANK function in our formula. If there is no value in myObject.Field_A__c then our condition is true and line 8 will get evaluated. On line 8 we store a value of 0 in our Integer variable called “a” — if there was indeed a value in myObject.Field_A__c then our condition would be false and therefore our “else {…}” block would get evaluated and the code on line 10 would tell our Integer variable “a” to hold onto the value of 1.
Lines 13-32: We repeat this logic for all four fields either storing 0 or 1 in each of our variables. When we are done we should have four variables that we can then add the values they hold together like we do on line 31. But that line looks just a bit different doesn’t it? Here we are saying “Hey compiler, remember that Boolean variable I told you about? Well take the value of adding a + b + c + d (my picture left out the + d — sorry) and compare that to see if its greater than 1 and store that result in my isGreater variable.” That may be a bit much to grasp, and I get it. Here’s what is happening. We are adding (a + b + c + d) but much like the order of operations from elementary school, we want that math to happen before we compare it to 1. We could have written it like so:
Integer i = a + b + c + d;
isGreater = i > 1;
But we can put this all on one line. Remember whenever we do a comparison, we either get a TRUE or FALSE value and Boolean variables know how to hold on TRUE or FALSE values.
Lastly, we “return” the result of our comparison to whatever called it (again elsewhere in code). Our validation rule doesn’t have the concept of a “return value” per se — it just IS that result. (Essentially, technically it does “return a value” but you as the admin are shielded from all of that).
Now some of you out there are saying, “WOW that’s quite a bit of code when my formula was only 4 lines long!” and you’d be right, but formulas have some built in magic that hide some of that extra “stuff” from you. Also, remember I said there may be better ways of doing things? Not all code would have to be that long. This next snippet — don’t worry if you don’t get it, its only here for an example and to show you that not all code is so drawn out — would do essentially the same thing:

That above snippet has some more advanced things in them like looping over a list of items, etc. Perhaps we will eventually get there with this series. I’m not sure if we will have Part 2 next week — hopefully so, but it depends on an submissions I may get from readers, or perhaps SteveMo has some more gems hiding somewhere. So feel free to send me your ideas and keep this going. Let me know if you have questions or if I can help clarify anything else in this lesson.
:wq!